Bug report
I have this a test org and it's returning results fine using python
https://gist.github.com/johndpope/4bdf87792c034fadb157766c702253d0
Describe the bug
When running same query
I get no results in swift version.
result: Optional(PostgREST.PostgrestResponse<()>(underlyingResponse: Get.Response<()>(value: (), response: <NSHTTPURLResponse: 0x600001263020> { URL: https://qfwzdkpmyzajmmvupgzy.supabase.co/rest/v1/tweets?select=* } { Status Code: 200, Headers {
"Access-Control-Allow-Origin" = (
"*"
);
"Alt-Svc" = (
"h3=\":443\"; ma=86400, h3-29=\":443\"; ma=86400"
);
"Content-Encoding" = (
br
);
"Content-Location" = (
"/tweets?select=%2A"
);
"Content-Type" = (
"application/json; charset=utf-8"
);
Date = (
"Thu, 17 Nov 2022 20:43:22 GMT"
);
Server = (
cloudflare
);
Vary = (
"Accept-Encoding"
);
Via = (
"kong/2.8.1"
);
"cf-cache-status" = (
DYNAMIC
);
"cf-ray" = (
"76bb4c4babd6a97a-SYD"
);
"content-profile" = (
public
);
"content-range" = (
"0-999/*"
);
"sb-gateway-version" = (
1
);
"x-kong-proxy-latency" = (
0
);
"x-kong-upstream-latency" = (
15
);
} }, data: 422860 bytes, task: LocalDataTask <47B6B060-DF8F-4921-AE38-CF831D362865>.<1>, metrics: Optional((Task Interval) <_NSConcreteDateInterval: 0x60000125f3e0> (Start Date) 2022-11-17 20:43:20 +0000 + (Duration) 2.450190 seconds = (End Date) 2022-11-17 20:43:22 +0000
(Redirect Count) 0
(Transaction Metrics) (Request) <NSMutableURLRequest: 0x60000101c320> { URL: https://qfwzdkpmyzajmmvupgzy.supabase.co/rest/v1/tweets?select=* }
(Response) <NSHTTPURLResponse: 0x600001262240> { URL: https://qfwzdkpmyzajmmvupgzy.supabase.co/rest/v1/tweets?select=* } { Status Code: 200, Headers {
"Access-Control-Allow-Origin" = (
"*"
);
"Alt-Svc" = (
"h3=\":443\"; ma=86400, h3-29=\":443\"; ma=86400"
);
"Content-Encoding" = (
br
);
"Content-Location" = (
"/tweets?select=%2A"
);
"Content-Type" = (
"application/json; charset=utf-8"
);
Date = (
"Thu, 17 Nov 2022 20:27:54 GMT"
);
Server = (
cloudflare
);
Vary = (
"Accept-Encoding"
);
Via = (
"kong/2.8.1"
);
"cf-cache-status" = (
DYNAMIC
);
"cf-ray" = (
"76bb35a7adf3a974-SYD"
);
"content-profile" = (
public
);
"content-range" = (
"0-999/*"
);
"sb-gateway-version" = (
1
);
"x-kong-proxy-latency" = (
1
);
"x-kong-upstream-latency" = (
15
);
} }
(Fetch Start) 2022-11-17 20:43:20 +0000
(Domain Lookup Start) (null)
(Domain Lookup End) (null)
(Connect Start) (null)
(Secure Connection Start) (null)
(Secure Connection End) (null)
(Connect End) (null)
(Request Start) 2022-11-17 20:43:20 +0000
(Request End) 2022-11-17 20:43:20 +0000
(Response Start) 2022-11-17 20:43:20 +0000
(Response End) 2022-11-17 20:43:20 +0000
(Protocol Name) (null)
(Proxy Connection) NO
(Reused Connection) NO
(Fetch Type) Local Cache
(Request Header Bytes) 0
(Request Body Transfer Bytes) 0
(Request Body Bytes) 0
(Response Header Bytes) 0
(Response Body Transfer Bytes) 0
(Response Body Bytes) 0
(Local Address) (null)
(Local Port) (null)
(Remote Address) (null)
(Remote Port) (null)
(TLS Protocol Version) 0x0000
(TLS Cipher Suite) 0x0000
(Cellular) NO
(Expensive) NO
(Constrained) NO
(Multipath) NO
(Request) <NSURLRequest: 0x60000101caf0> { URL: https://qfwzdkpmyzajmmvupgzy.supabase.co/rest/v1/tweets?select=* }
(Response) <NSHTTPURLResponse: 0x60000124e260> { URL: https://qfwzdkpmyzajmmvupgzy.supabase.co/rest/v1/tweets?select=* } { Status Code: 200, Headers {
"Access-Control-Allow-Origin" = (
"*"
);
"Alt-Svc" = (
"h3=\":443\"; ma=86400, h3-29=\":443\"; ma=86400"
);
"Content-Encoding" = (
br
);
"Content-Location" = (
"/tweets?select=%2A"
);
"Content-Type" = (
"application/json; charset=utf-8"
);
Date = (
"Thu, 17 Nov 2022 20:43:22 GMT"
);
Server = (
cloudflare
);
Vary = (
"Accept-Encoding"
);
Via = (
"kong/2.8.1"
);
"cf-cache-status" = (
DYNAMIC
);
"cf-ray" = (
"76bb4c4babd6a97a-SYD"
);
"content-profile" = (
public
);
"content-range" = (
"0-999/*"
);
"sb-gateway-version" = (
1
);
"x-kong-proxy-latency" = (
0
);
"x-kong-upstream-latency" = (
15
);
} }
(Fetch Start) 2022-11-17 20:43:20 +0000
(Domain Lookup Start) 2022-11-17 20:43:20 +0000
(Domain Lookup End) 2022-11-17 20:43:20 +0000
(Connect Start) 2022-11-17 20:43:20 +0000
(Secure Connection Start) 2022-11-17 20:43:20 +0000
(Secure Connection End) 2022-11-17 20:43:20 +0000
(Connect End) 2022-11-17 20:43:20 +0000
(Request Start) 2022-11-17 20:43:20 +0000
(Request End) 2022-11-17 20:43:20 +0000
(Response Start) 2022-11-17 20:43:22 +0000
(Response End) 2022-11-17 20:43:22 +0000
(Protocol Name) h3
(Proxy Connection) NO
(Reused Connection) NO
(Fetch Type) Network Load
(Request Header Bytes) 998
(Request Body Transfer Bytes) 0
(Request Body Bytes) 0
(Response Header Bytes) 295
(Response Body Transfer Bytes) 110951
(Response Body Bytes) 422860
(Local Address) 192.168.1.100
(Local Port) 62732
(Remote Address) 104.18.26.135
(Remote Port) 443
(TLS Protocol Version) 0x0304
(TLS Cipher Suite) 0x1301
(Cellular) NO
(Expensive) NO
(Constrained) NO
(Multipath) NO
)), count: nil))
To Reproduce
override func viewDidLoad() {
super.viewDidLoad()
setupDatabase()
}
func setupDatabase() {
let SUPABASE_URL = URL(string:"https://qfwzdkpmyzajmmvupgzy.supabase.co")!
let ANON_KEY = "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9.eyJpc3MiOiJzdXBhYmFzZSIsInJlZiI6InFmd3pka3BteXpham1tdnVwZ3p5Iiwicm9sZSI6ImFub24iLCJpYXQiOjE2NjgxMzAzMjYsImV4cCI6MTk4MzcwNjMyNn0.kEweOMrRSJ0mtOIXnsRmbNxf4yqoZAZf398jlGgSxtQ"
self.client = SupabaseClient(supabaseURL: SUPABASE_URL, supabaseKey: ANON_KEY)
Task {
await fetchRows();
}
}
func fetchRows() async {
let result = try? await self.client?.database.from("tweets").select(columns: "*").execute();
print("result:",result.debugDescription);
}
Steps to reproduce the behavior, please provide code snippets or a repository:
I'm on release-candidate branch
Expected behavior
running python version - I get results back
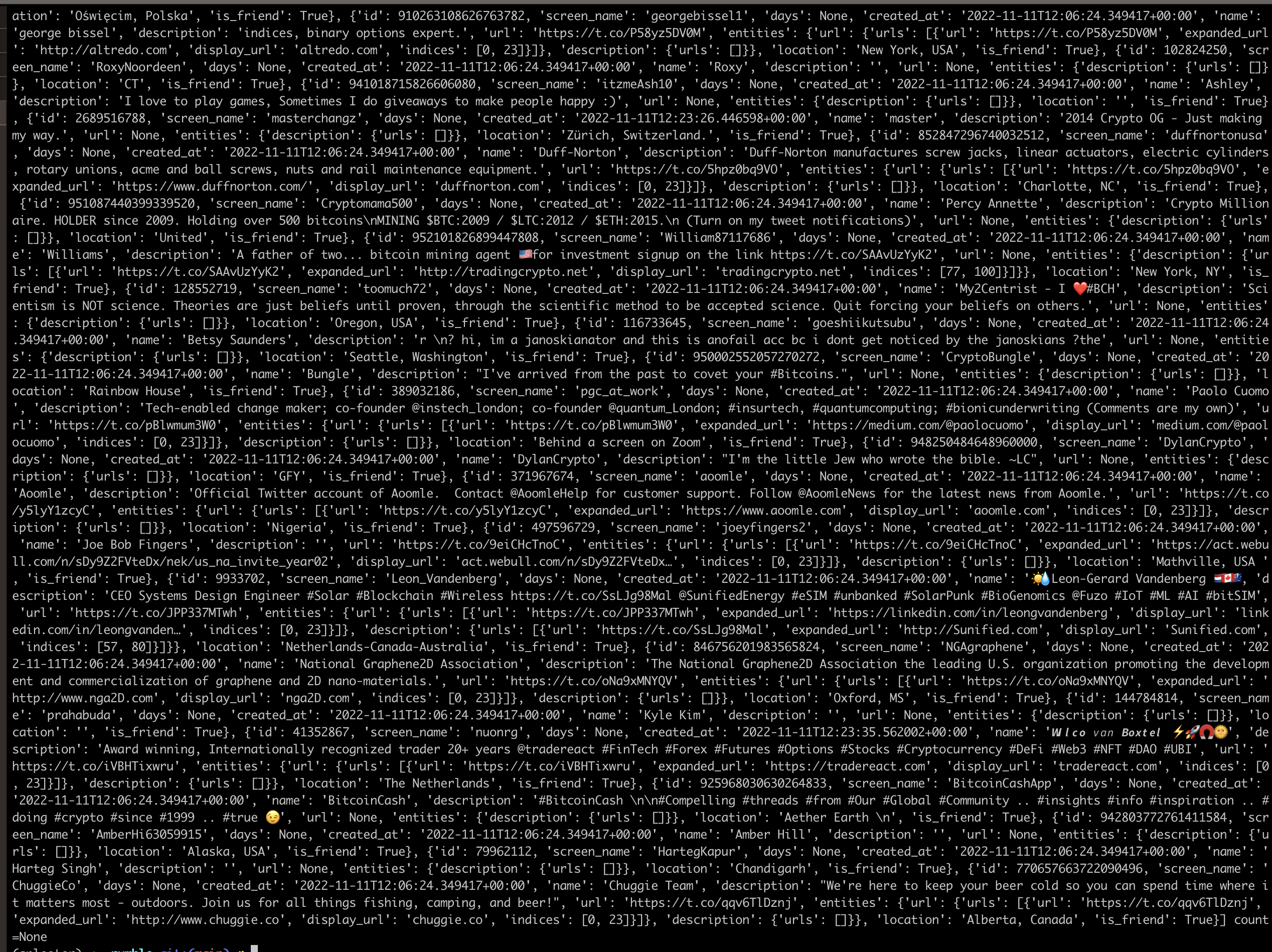
but on ios - I get results = nil
Screenshots
If applicable, add screenshots to help explain your problem.
System information
- OS: macos - ventura
- Version of Node.js: swift release candidate branch 0.0.9
Additional context
I've successfully used and got data from superbase in another project - not sure what's going on here.
maybe to do with async stuff?
bug