EFQRCode is a lightweight, pure-Swift library for generating stylized QRCode images with watermark or icon, and for recognizing QRCode from images, inspired by qrcode. Based on CoreGraphics
, CoreImage
, and ImageIO
, EFQRCode provides you a better way to handle QRCode in your app, no matter if it is on iOS, macOS, watchOS, and/or tvOS. You can integrate EFQRCode through CocoaPods, Carthage, and/or Swift Package Manager.
Examples
![]() |
![]() |
![]() |
![]() |
---|---|---|---|
![]() |
![]() |
![]() |
![]() |
Demo Projects
TestFlight
You can click the TestFlight
button below to download demo with TestFlight:
App Store
You can click the App Store
button below to download demo, support iOS, tvOS and watchOS:
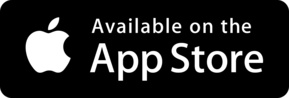
You can also click the Mac App Store
button below to download demo for macOS:
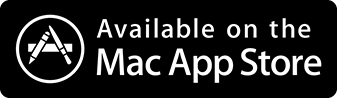
Compile Demo Manually
To run the example project manually, clone the repo, demos are in the 'Examples' folder, remember run command sh Startup.sh
in terminal to get all dependencies first, then open EFQRCode.xcworkspace
with Xcode and select the target you want, run.
Or you can run the following command in terminal:
git clone [email protected]:EFPrefix/EFQRCode.git; cd EFQRCode; sh Startup.sh; open 'EFQRCode.xcworkspace'
Requirements
Version | Needs |
---|---|
1.x | Xcode 8.0+ Swift 3.0+ iOS 8.0+ / macOS 10.11+ / tvOS 9.0+ |
4.x | Xcode 9.0+ Swift 4.0+ iOS 8.0+ / macOS 10.11+ / tvOS 9.0+ / watchOS 2.0+ |
5.x | Xcode 11.1+ Swift 5.0+ iOS 8.0+ / macOS 10.11+ / tvOS 9.0+ / watchOS 2.0+ |
6.x | Xcode 12.0+ Swift 5.1+ iOS 9.0+ / macOS 10.10+ / tvOS 9.0+ / watchOS 2.0+ |
Installation
CocoaPods
EFQRCode is available through CocoaPods. To install it, simply add the following line to your Podfile:
pod 'EFQRCode', '~> 6.1.0'
Then, run the following command:
$ pod install
Carthage
IMPORTANT: this workaround is necessary for Carthage to somewhat work in Xcode 12.
Carthage is a decentralized dependency manager that builds your dependencies and provides you with binary frameworks.
You can install Carthage with Homebrew using the following command:
$ brew update
$ brew install carthage
To integrate EFQRCode into your Xcode project using Carthage, specify it in your Cartfile
:
github "EFPrefix/EFQRCode" ~> 6.1.0
Run carthage update
to build the framework and drag the built EFQRCode.framework
into your Xcode project.
Swift Package Manager
The Swift Package Manager is a tool for automating the distribution of Swift code and is integrated into the Swift compiler.
Once you have your Swift package set up, adding EFQRCode as a dependency is as easy as adding it to the dependencies
value of your Package.swift
.
dependencies: [
.package(url: "https://github.com/EFPrefix/EFQRCode.git", .upToNextMinor(from: "6.1.0"))
]
Quick Start
1. Import EFQRCode
Import EFQRCode module where you want to use it:
import EFQRCode
2. Recognition
A String Array is returned as there might be several QR Codes in a single CGImage
:
if let testImage = UIImage(named: "test.png")?.cgImage {
let codes = EFQRCode.recognize(testImage)
if !codes.isEmpty {
print("There are \(codes.count) codes")
for (index, code) in codes.enumerated() {
print("The content of QR Code \(index) is \(code).")
}
} else {
print("There is no QR Codes in testImage.")
}
}
3. Generation
Create QR Code image, basic usage:
Parameter | Description |
---|---|
content |
REQUIRED, content of QR Code |
size |
Width and height of image |
backgroundColor |
Background color of QRCode |
foregroundColor |
Foreground color of QRCode |
watermark |
Background image of QRCode |
if let image = EFQRCode.generate(
for: "https://github.com/EFPrefix/EFQRCode",
watermark: UIImage(named: "WWF")?.cgImage
) {
print("Create QRCode image success \(image)")
} else {
print("Create QRCode image failed!")
}
Result:
4. Generation from GIF
Use EFQRCode.generateGIF
to create GIF QRCode.
Parameter | Description |
---|---|
generator |
REQUIRED, an EFQRCodeGenerator instance with other settings |
data |
REQUIRED, encoded input GIF |
delay |
Output QRCode GIF delay, emitted means no change |
loopCount |
Times looped in GIF, emitted means no change |
if let qrCodeData = EFQRCode.generateGIF(
using: generator, withWatermarkGIF: data
) {
print("Create QRCode image success.")
} else {
print("Create QRCode image failed!")
}
You can get more information from the demo, result will like this:
5. Next
Learn more from User Guide.
Recommendations
- Please select a high contrast foreground and background color combinations;
- To improve the definition of QRCode images, increase
size
, or scale up usingmagnification
(instead); - Magnification too high/size too large/contents too long may cause failure;
- It is recommended to test the QRCode image before put it into use;
- You can contact me if there is any problem, both
Issue
andPull request
are welcome.
PS of PS: I wish you can click the Star
button if this tool is useful for you, thanks, QAQ...
Other Platforms/Languages
Platforms/Languages | Link |
---|---|
Objective-C | https://github.com/z624821876/YSQRCode |
Java | https://github.com/SumiMakito/AwesomeQRCode |
JavaScript | https://github.com/SumiMakito/Awesome-qr.js |
Kotlin | https://github.com/SumiMakito/AwesomeQRCode-Kotlin |
Python | https://github.com/sylnsfar/qrcode |
Contributors
This project exists thanks to all the people who contribute. [Contribute]
PS: We use QRCodeSwift to generate QR code on watchOS, thanks to ApolloZhu.
Donations
If you think this project has brought you help, you can buy me a cup of coffee. If you like this project and are willing to provide further support for it's development, you can choose to become Backer
or Sponsor
in Open Collective.
Backers
Thank you to all our backers!
Sponsors
Support this project by becoming a sponsor. Your logo will show up here with a link to your website. [Become a sponsor]
Thanks for your support,
Thanks
- Thanks for the help from JetBrains's Open Source Support Program.
Awards
![]() |
![]() |
Apps using EFQRCode
|
|
|
|
|
|
|
|
|
|
|
|
Other
Part of the pictures in the demo project and guide come from the internet. If there is any infringement of your legitimate rights and interests, please contact us to delete.
Contact
Email: [email protected]
License
EFQRCode is available under the MIT license. See the LICENSE file for more info.